前端学习-React
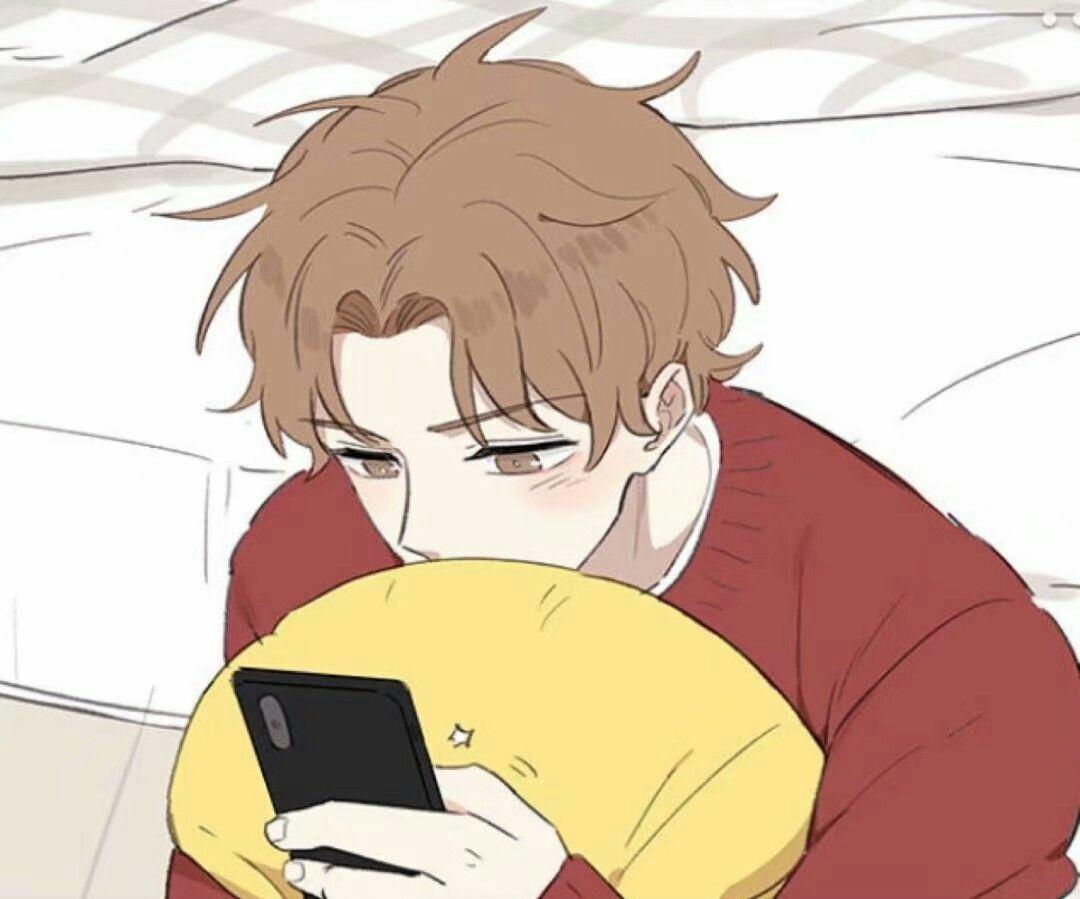
学习React和状态管理工具Redux等一些笔记
1. Redux
1.1. 基本概念
Redux 是JavaScript的一种预测性状态容器,用于构建一致性的应用。包含以下重要概念:
- Store: 所有的状态都被保存在一个称为Store的对象中
- State: 应用的状态,保存在Store中,Store是只读的,只能通过dispatch(发送)一个Action来改变
- Action: 带有type属性的JavaScript对象,用于描述发生什么
- Reducer: 接受当前的State和一个Action,返回一个新的State。在Redux中,所有状态更新逻辑集中在Reducer中。
1.2. 工作流程
- 首先,用户(或者其他事件源)会触发一个 Action。
- 然后,这个 Action 会被发送(dispatch)到 Store。
- Store 将当前的 State 和这个 Action 一起传递给 Reducer。
- Reducer 会根据这个 Action 的
type
,计算出一个新的 State。 - 最后,Store 将这个新的 State 保存起来。如果有任何视图或者组件依赖这个 State,它们会自动更新。
1.3. Slice
一个Slice是Redux状态树的一部分,一般每一个模块有一个slice,用于管理这个模块的状态更新。Redux Toolkit提供了一个createSlice函数,可以帮助快速创建slice,接收一个配置对象,包括slice的名字、初始状态和一些reducer函数,不同的reducer函数用于处理不同的action,并返回新的状态。一个example如下:
1 | import { createSlice } from '@reduxjs/toolkit' |
1.4. Redux Toolkit
Redux Toolkit 作为Redux团队官方的一个工具库,主要提供以下功能:
- configureStore: 这是一个创建 Redux store 的函数,它自动集成了 Redux DevTools 扩展,并自动添加了一些常用的 Redux 中间件,如 Redux Thunk。
- createReducer 和 createSlice: 这两个函数可以帮助你更方便地创建 reducer。特别是
createSlice
,它可以一次性创建 action creator 和 reducer,且无需手动编写 action type,大大简化了 Redux 的使用。 - createAsyncThunk: 这个函数用于处理异步逻辑。你只需要提供一个执行异步操作的函数,
createAsyncThunk
会自动创建对应的 action,并处理 pending、fulfilled 和 rejected 三种状态。 - createEntityAdapter: 这个函数可以帮助你管理 Redux state 中的集合数据,提供了一些常用的操作函数,如排序、更新等。
2. props管理
props是组件之间交互的方式,是父组件向子组件传递数据的方式,可以将字符串、数字、对象数组甚至其他组件作为React组件。props是只读的,意味着不能在组件内部改变props的值。一个实例如下:
1 | import React, { useState } from 'react'; |
子组件定义了一个名为onlick的prop,像形式参数,handleClick是实际参数。
3. useState
一个内置Hook,允许在React函数组件中添加状态,接受一个参数,作为函数的初始值,返回一个数组,数组的两个元素为当前的状态值和更新状态的函数。以下是一个例子:
1 | import React, { useState } from 'react'; |
- Title: 前端学习-React
- Author: OnyYao
- Created at : 2023-03-17 00:00:00
- Updated at : 2025-01-14 16:24:19
- Link: https://www.onyyao.top/2023/03/17/前端学习-Redux/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments